While the Node.JS community is quickly evolving all the libraries and making your life as a developer easier every day. One thing you read little about, is the development environment. Every one uses their own cobbled together thing. To get you started with Node.JS, this post is a step by step guide to creating a full working and reproducible environment that supports deployment to Heroku.
I’ve previously sung praise for VirtualBox. It’s a tool that any developer should have. It will allow you to create an environment that’s easily portable and can be quickly reproduced by any new developer on the team.
Step 1. Installing the Operating System
If you’ve previously created a Linux virtual machine with VirtualBox, you can probably skip this step. But for those new and for my own reference, here are my steps:
- Download the latest ISO image of your prefered Linux. I usually just go for Ubuntu because it has the largest community and thus the most chance any issues you may encounter have already been solved.
- Create a new virtual machine, everything is default, except
- mount the Ubuntu ISO image on the CD-ROM controller.
- create a new automounted shared folder (handy for sending files between both operating systems)
- 1024 MB of memory is plenty. 512 works too, but it’s a little more comfortable with some more.
- the 8GB hard drive space is plenty for all of Node, an Apache server and one or two database engines. If you are going to install anything more, you’re either going to have to be careful with space (like removing Libre Office), or just make the virtual hard drive 16GB.
- Start the new VM
- If the CD image is mounted, it should automatically start the Ubuntu installation. This is going to take some time, so grab a coffee.
- After your first boot in your newly installed VM, first open the update manager and update everything. At least if you intend on keeping your VM up-to-date, if not, it’s probably best to disable to automated update checks.
- For Heroku, Github, etc access, you should also generate a public/private key pair:
- ssh-keygen -t rsa
- Feel free to install any editor you like. Depending on my mood, I use VIM or Geany.
Step 2. Integrating Guest and Host
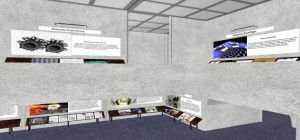
Integration between the Ubuntu guest VM and your host will allow you a few nice perks, like being able to use your full screen, exchange files and even seamlessly integrate both systems (if you like this, it’s not for every one)
- Install the VirtualVox Guest Additions from the VirtualBox menu that shows up when your VM is running. Or you can press Host-D (the Host key is associated with the right ctrl button on Windows)
- With those additions installed, the shared folder will be mounted. Now you just need to add your user to the correct usergroup so he has access. Open a terminal and type (on the Ubuntu VM):
- sudo usermod -a -G vboxsf
- now logout and log back on
- test your share access with “ls /media/sf_
”
- sudo usermod -a -G vboxsf
Step 3. Setting Up and Configuring Node.JS and Heroku
Now we’re getting close to getting some actual work done. If you don’t already have a Heroku account and want to follow along, now is the time to sign up.
- You can compile Node.JS for yourself. It is a pretty painless operation, but via the Ubuntu (well, Debian) package manager it’s even easier:
- sudo apt-get install python-software-properties
- sudo apt-add-repository ppa:chris-lea/node.js
- sudo apt-get update
- sudo apt-get install nodejs npm
- sudo apt-get install nodejs-dev (Needed for some of the database drivers)
- Test out your install by creating a helloworld.js that contains:
- console.log(‘hello world’)
- Run: node helloworld.js
- Install the Heroku toolbelt
- Check the correct installation by login in and adding your key:
- heroku login
- heroku keys:add ~/.ssh/id_rsa.pub
- And configure your default git e-mail and name:
- git config –global user.name “
” - git config –global user.email “
”
- git config –global user.name “
Step 4. Creating a Minimal CRUD App
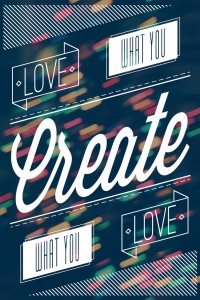
You’re now ready to start developing and deploying your Node.JS application. I like to use RailwayJS to get a head start, but there are many many other options.
- sudo npm install railway -g
- sudo npm install coffee-script -g (avoids having to download this for every railway applications)
- railway init blog && cd blog
- npm install -l
- railway generate crud post title content
- railway server 8888
- open http://127.0.0.1:8888/posts
This will set you up with a minimal CRUD application that uses an in-memory store for all storage.
Step 5. Configuring & Deploying to Heroku
Before deploying, there are a few steps and tweaks you will want to make.
Adding a Redis session store:
-
sudo apt-get install redis-server
- add”connect-heroku-redis” : “>= 0.1.2” in your package.json
- in the config/environment.js file:
- HerokuRedisStore = require(‘connect-heroku-redis’)(express);
-
app.use(express.session({secret: ‘secret’, store: new HerokuRedisStore }));
- You may want to replace the secret!
Make sure you are using the latest Node version on Heroku, by adding the following to your package.json:
-
“engines” : { “node” : “0.6.x” }
You probably want to have git ignore the node_modules folder:
-
echo node_modules > .gitignore
Set up Heroku as the production environment:
-
heroku config:add NODE_ENV=production
- But disable file logging in the Railwayjs production.js file: remove the line “app.settings.quiet=true”
I’m keeping the database configuration for another post, because there’s just so much choice. But both MongoDB and PostgreSQL can be tested out for free on Heroku and are fairly easy to configure.
The actual deployment looks like this:
- git init
- git add .
- git commit -m “first version”
- heroku create –stack cedar
- heroku addons:add redistogo:nano
- git push heroku master
- heroku ps:scale web=1
- heroku open
This should bring up a browser with your deployed application.
During actual development the deployment flow is as simple as:
- git add
- git commit -m “commit message”
- git push heroku master
Conclusion
A reliable development environment is the best guarantee that you’ll not only experiment with Node.JS, but also actually get some work done. Developing in Node will bring back the programmer’s joy you felt before enormous frameworks and gigantic API’s.